XML(eXtensible Markup Language)は、ウェブサイトやアプリケーションでデータを構造化するためのマークアップ言語です。XMLファイルはテキストベースで、タグを使用してデータを示します。XMLは、異なるプログラミング言語やプラットフォーム間でデータを交換するために広く使用されています。本記事では、PythonによるXMLファイルに含まれる情報を取得する/使用する方法を紹介します。
下記の様な内容で悩んでいる/困っている場合に使える方法を参考までにご共有させて頂きます。
・Pythonの場合、どうやってXMLファイルを使うの?
・Pythonのリスト(配列)は、どの様にXMLファイルを扱うのだろうか?
目次
XMLファイルに含まれる情報の取得/使用(自作関数)
関数の定義(読み込み用の関数)
def readFileXML(input_path: str) -> tuple:
### tree: <class 'xml.etree.ElementTree.ElementTree'>
### root: <class 'xml.etree.ElementTree.Element'>
tree = ET.parse(input_path)
root = tree.getroot()
return (tree, root)
関数の定義(表示用の関数)
def xmlPrintXMLElement(input_node, input_str:str="node"):
print()
print(f">>> Node Information:")
print(f"{input_str} >> Tag: {repr(input_node.tag)}")
print(f"{input_str} >> Attribute: {input_node.attrib}")
print(f"{input_str} >> Text: {repr(input_node.text)}")
print(f"{input_str} >> SubElement >> Tag:")
print(f"{[node.tag for node in input_node]}")
print()
使用例
データの内容(sample.xml)
<?xml version="1.0"?>
<data>
<country name="Liechtenstein">
<rank>1</rank>
<year>2008</year>
<gdppc>141100</gdppc>
<neighbor name="Austria" direction="E" />
<neighbor name="Switzerland" direction="W" />
</country>
<country name="Singapore">
<rank>4</rank>
<year>2011</year>
<gdppc>59900</gdppc>
<neighbor name="Malaysia" direction="N" />
</country>
<country name="Panama">
<rank>68</rank>
<year>2011</year>
<gdppc>13600</gdppc>
<neighbor name="Costa Rica" direction="W" />
<neighbor name="Colombia" direction="E" />
</country>
</data>
プログラムの内容
input_file_path = "INPUT/_Python_XMLExample/sample001.xml"
tree, root = readFileXML(input_file_path)
xmlPrintXMLElement(root, "root")
node_0 = root[0]
xmlPrintXMLElement(node_0, "node_0")
node_0 = [node for node in root if (node.tag=="country") and (node.get("name")=="Liechtenstein")][0]
xmlPrintXMLElement(node_0, "node_0")
node_0_0 = node_0[0]
xmlPrintXMLElement(node_0_0, "node_0_0")
node_0_0 = node_0.find("rank")
xmlPrintXMLElement(node_0_0, "node_0_0")
print(f"{root.tag = }")
print(f"{node_0.get('name') = }")
print(f"{node_0.attrib.get('name') = }")
print(f"{node_0.attrib['name'] = }")
print(f"{node_0_0.text = }")
>>> Node Information:
root >> Tag: 'data'
root >> Attribute: {}
root >> Text: '\n '
root >> SubElement >> Tag:
['country', 'country', 'country']
>>> Node Information:
node_0 >> Tag: 'country'
node_0 >> Attribute: {'name': 'Liechtenstein'}
node_0 >> Text: '\n '
node_0 >> SubElement >> Tag:
['rank', 'year', 'gdppc', 'neighbor', 'neighbor']
>>> Node Information:
node_0 >> Tag: 'country'
node_0 >> Attribute: {'name': 'Liechtenstein'}
node_0 >> Text: '\n '
node_0 >> SubElement >> Tag:
['rank', 'year', 'gdppc', 'neighbor', 'neighbor']
>>> Node Information:
node_0_0 >> Tag: 'rank'
node_0_0 >> Attribute: {}
node_0_0 >> Text: '1'
node_0_0 >> SubElement >> Tag:
[]
>>> Node Information:
node_0_0 >> Tag: 'rank'
node_0_0 >> Attribute: {}
node_0_0 >> Text: '1'
node_0_0 >> SubElement >> Tag:
[]
root.tag = 'data'
node_0.get('name') = 'Liechtenstein'
node_0.attrib.get('name') = 'Liechtenstein'
node_0.attrib['name'] = 'Liechtenstein'
node_0_0.text = '1'
Pythonで取り扱うXMLデータの内容
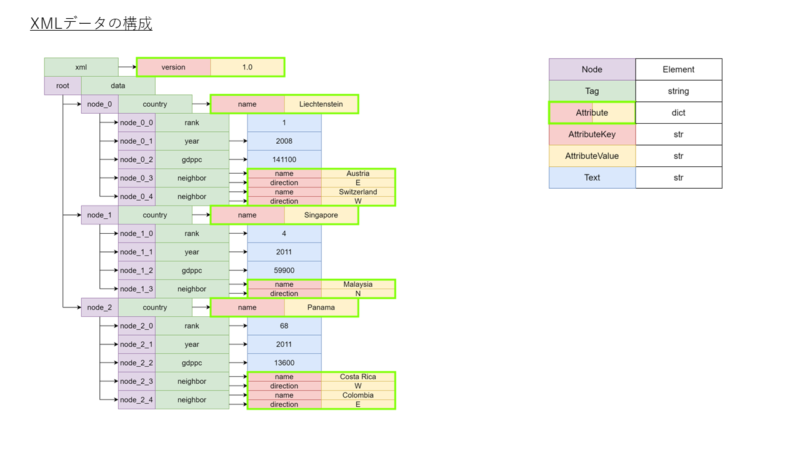
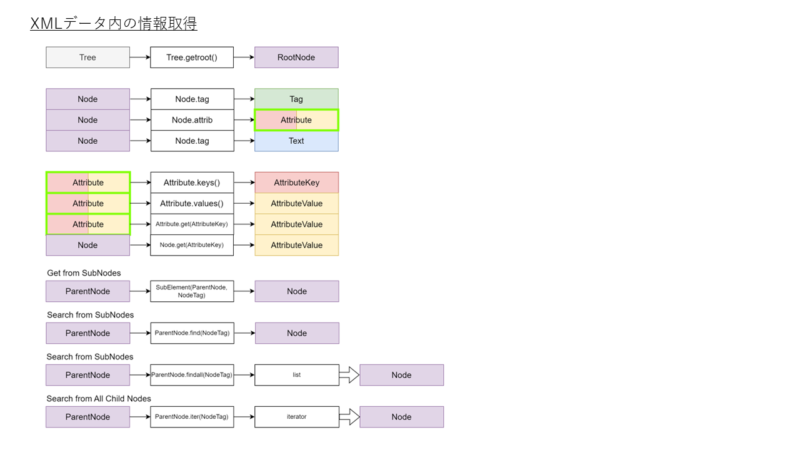
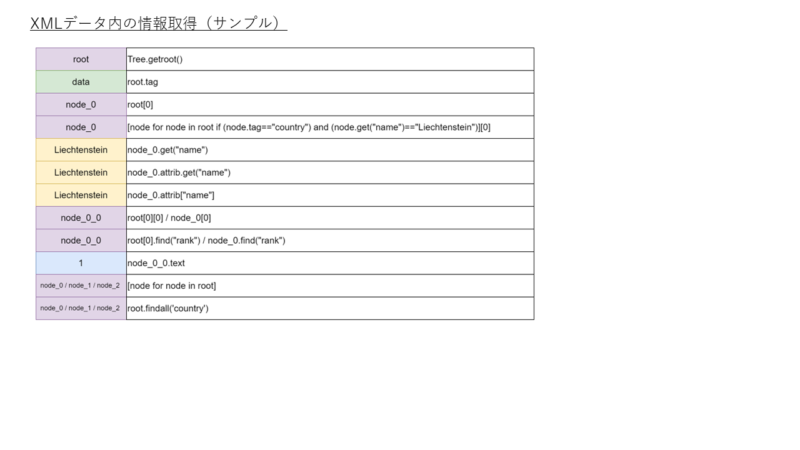
まとめ
Pythonはデータ活用する上で、とても便利なプログラミング言語です。この記事で紹介させて頂いた方法で「XMLファイル」に格納されている情報を読み込み、データ処理/データ分析/データ管理等でぜひ活用してみてください。
関連検索ワード
How to get/use a XML data with Python?
関連キーワード
python, 入門, 初心者, ファイル, 取得, 使用, XML, file, get, use