JSON(JavaScript Object Notation)は、データを表現するための軽量なデータ形式です。JavaScriptのオブジェクト表記法を基にしており、テキスト形式でデータを表現します。JSONは、ウェブアプリケーションやウェブサービスでデータの交換や保存に広く利用されています。本記事では、PythonによるJSONファイルに含まれる情報を取得する/使用する方法を紹介します。
下記の様な内容で悩んでいる/困っている場合に使える方法を参考までにご共有させて頂きます。
・Pythonの場合、どうやってJSONファイルを使うの?
・Pythonのリスト(配列)は、どの様にJSONファイルを扱うのだろうか?
目次
JSONファイルに含まれる情報の取得/使用(自作関数)
関数の定義
def readFileJSON(input_path: str, input_encoding: str = "utf_8") -> dict:
file_data = open(input_path, mode='r', encoding=input_encoding)
return json.load(file_data)
使用例
データの内容(sample.json)
{
"string": {
"EN": "one",
"JP": "いち"
},
"number": [
1,
1.23,
1e+23
],
"etc": [
true,
false,
null
]
}
プログラムの内容
input_file_path = "INPUT/sample.json"
json_data = readFileJSON(input_file_path)
print(f"object_0:\n{json_data} {type(json_data)}\n")
object_1 = json_data["string"]
print(f"object_1:\n{object_1} {type(object_1)}\n")
array_0 = json_data["number"]
print(f"array_0:\n{array_0} {type(array_0)}\n")
array_1 = json_data["etc"]
print(f"array_1:\n{array_1} {type(array_1)}\n")
print(f"{object_1['EN'] = }")
print(f"{object_1['JP'] = }")
print(f"{array_0[0] = }")
print(f"{array_0[1] = }")
print(f"{array_0[2] = }")
print(f"{array_1[0] = }")
print(f"{array_1[1] = }")
print(f"{array_1[2] = }")
object_0:
{'string': {'EN': 'one', 'JP': 'いち'}, 'number': [1, 1.23, 1e+23], 'etc': [True, False, None]} <class 'dict'>
object_1:
{'EN': 'one', 'JP': 'いち'} <class 'dict'>
array_0:
[1, 1.23, 1e+23] <class 'list'>
array_1:
[True, False, None] <class 'list'>
object_1['EN'] = 'one'
object_1['JP'] = 'いち'
array_0[0] = 1
array_0[1] = 1.23
array_0[2] = 1e+23
array_1[0] = True
array_1[1] = False
array_1[2] = None
Pythonで取り扱うJSONデータの内容
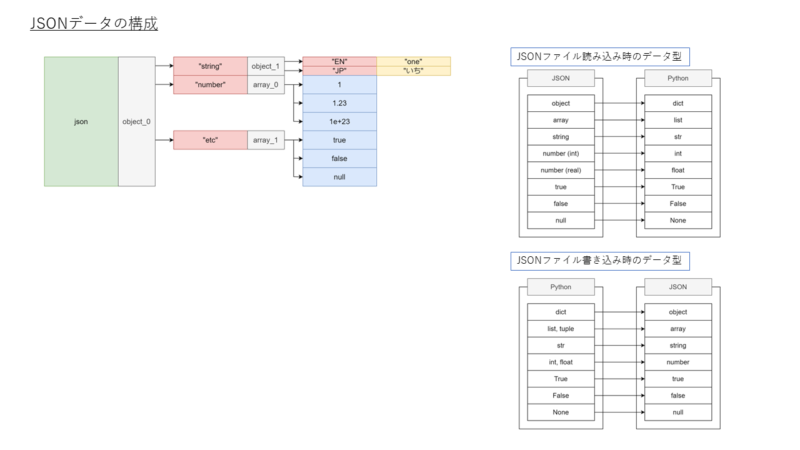
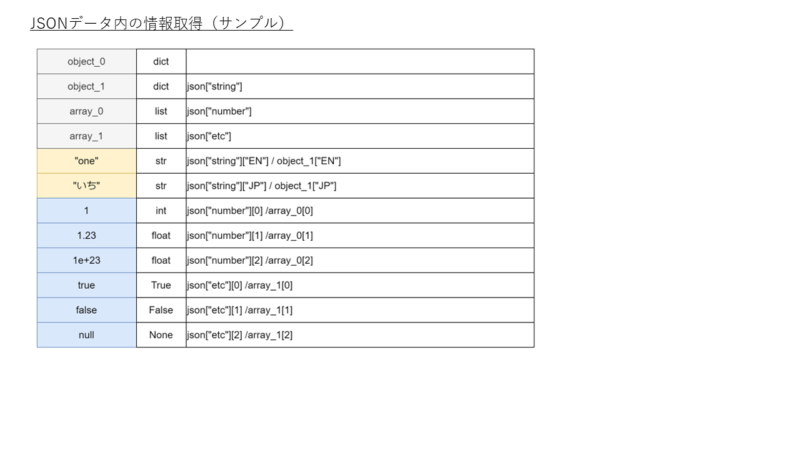
まとめ
Pythonはデータ活用する上で、とても便利なプログラミング言語です。この記事で紹介させて頂いた方法で「JSONファイル」に格納されている情報を読み込み、データ処理/データ分析/データ管理等でぜひ活用してみてください。
関連検索ワード
How to get/use a JSON data with Python?
関連キーワード
python, 入門, 初心者, ファイル, 取得, 使用, JSON, file, get, use