「TXT」ファイルをPythonで読み込み, 「LIST」として格納する方法についての記事となっております。
データ構造
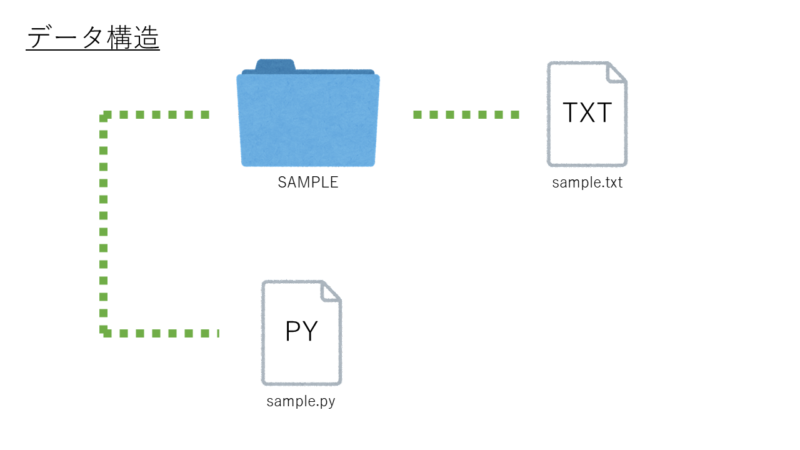
### Data Structure
### - SAMPLE
### - sample.txt
### - sample.py
処理の流れ
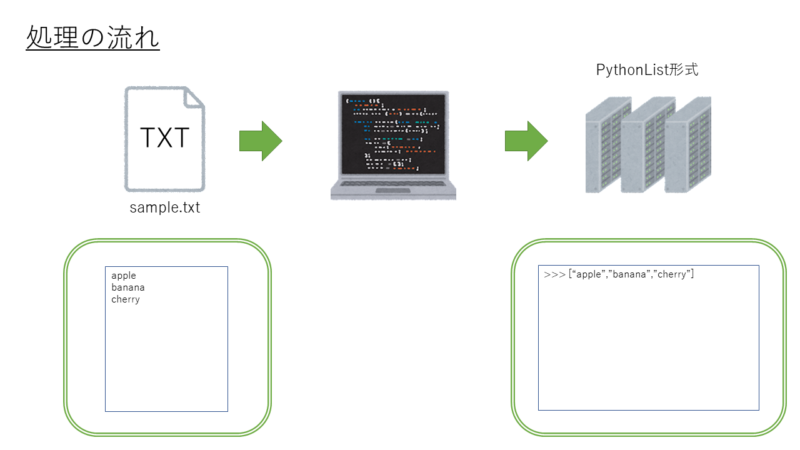
実行ファイルの内容
apple
banana
cherry
def option_001(filePath):
file_IO = open(filePath)
info_STR = file_IO.read()
info_LIST = info_STR.split()
### print(info_STR)
### apple
### banana
### cherry
### print(info_LIST)
### ['apple', 'banana', 'cherry']
file_IO.close()
return info_LIST
def option_002(filePath):
with open(filePath) as file_IO:
info_STR = file_IO.read()
info_LIST = info_STR.split()
### print(info_STR)
### apple
### banana
### cherry
### print(info_LIST)
### ['apple', 'banana', 'cherry']
return info_LIST
def option_003(filePath):
with open(filePath) as file_IO:
info_LIST = file_IO.readlines()
### print(info_LIST)
### ['apple\n', 'banana\n', 'cherry']
info_mod_LIST = [ info_STR.strip() for info_STR in info_LIST]
### print(info_mod_LIST)
### ['apple', 'banana', 'cherry']
return info_mod_LIST
def option_004(filePath):
info_LIST = []
with open(filePath) as file_IO:
while True:
info_STR = file_IO.readline()
if not info_STR: break
else:
### print(repr(info_STR))
info_LIST.append(info_STR.strip())
### 'apple\n'
### 'banana\n'
### 'cherry'
### print(info_LIST)
### ['apple', 'banana', 'cherry']
return info_LIST
def option_005(filePath):
info_LIST = []
with open(filePath) as file_IO:
for info_STR in file_IO:
### print(repr(info_STR))
info_LIST.append(info_STR.strip())
### 'apple\n'
### 'banana\n'
### 'cherry'
### print(info_LIST)
### ['apple', 'banana', 'cherry']
return info_LIST
### INPUT: File Path (str)
### OUTPUT: File Information (list)
FILE_PATH = "SAMPLE/sample.txt"
print(option_001(FILE_PATH))
print(option_002(FILE_PATH))
print(option_003(FILE_PATH))
print(option_004(FILE_PATH))
print(option_005(FILE_PATH))