こちらは「dict」(Python組み込み型)のサンプルコードについての記事となっております。
目次
基本情報 … Basic Information
Pythonの「dict」は、キーと値のペアを持つ、可変長のデータ型です。辞書は、キーを使用して値にアクセスできるハッシュテーブルのようなもので、高速な検索が可能です。キーはイミュータブルな型である必要がありますが、値には何でも使えます。例えば、文字列、数値、リスト、タプル、セット、その他の辞書、さらには関数なども値にすることができます。辞書は波括弧 {} で囲まれたキーと値のペアの集合で表現されます。
チートシート … Cheat Sheet
Create a Empty Dict | a = {} | {} | |
Create a Empty Dict | a = dict() | {} | |
Create a Dict | a = {1:”one”,2:”two”,3:”three”} | {1: ‘one’, 2: ‘two’, 3: ‘three’} | |
Create a Dict | a = dict(zip(list,list)) | {1: ‘one’, 2: ‘two’, 3: ‘three’} | |
Delete a Dict | del a | ||
Get a Length of the Dict | a=len(input_dict) | A. {} B. {1:”one”,2:”two”,3:”three”} | A. 0 B. 3 |
Get a Type of the Dict | a=type(input_dict) | A. {} B. {1:”one”,2:”two”,3:”three”} | A. <class ‘dict’> B. <class ‘dict’> |
Get a Value of the Dict with Key | a=input_dict[key] | A. {1:”one”,2:”two”,3:”three”}, 1 B. {1:”one”,2:”two”,3:”three”}, 4 | A. one B. KeyError |
Get a Value of the Dict with Key | a=input_dict.get(key) | A. {1:”one”,2:”two”,3:”three”}, 1 B. {1:”one”,2:”two”,3:”three”}, 4 | A. one B. None |
Get a Value of the Dict with Key | a=input_dict.get(key,except) | {1:”one”,2:”two”,3:”three”}, 4, “N/A” | N/A |
Edit a Dict with Key, Value | input_dict[key] = new_val | A. {1:”one”,2:”two”,3:”three”}, 1, “hundred” B. {1:”one”,2:”two”,3:”three”}, 4, “hundred | A. {1: ‘hundred’, 2: ‘two’, 3: ‘three’} B. {1: ‘hundred’, 2: ‘two’, 3: ‘three’, 4:’hundred’} |
Delete Key and Value of Dict | input_dict.clear() | {1: ‘one’, 2: ‘two’, 3: ‘three’} | {} |
Create a Dict with the Dict Copy a Dict | a = input_dict.copy() | {1: ‘one’, 2: ‘two’, 3: ‘three’} | {1: ‘one’, 2: ‘two’, 3: ‘three’} |
Create a Dict with Iter | a = dict.fromkeys(iter) | [1,2,3] | {1: None, 2: None, 3: None} |
Create a Dict with Iter, Value | a = dict.fromkeys(iter,value) | A. [1,2,3], 0 B. (1,2,3), 0 | {1: 0, 2: 0, 3: 0} |
Edit a Dict with the Key Pop a Value of the Dict | a = input_dict.pop(key) *KeyError | A. {1:”one”,2:”two”,3:”three”}, 1 B. {1:”one”,2:”two”,3:”three”}, 4 | A. one B. KeyError |
Edit a Dict with the Key Pop a Value of the Dict | a = input_dict.pop(key,except) | {1:”one”,2:”two”,3:”three”}, 4, “N/A” | N/A |
Edit a Dict with Key | a = dict.setdefault(key) | A. {1:”one”,2:”two”,3:”three”}, 1 B. {1:”one”,2:”two”,3:”three”}, 4 | A. {1: ‘one’, 2: ‘two’, 3: ‘three’} B. {1: ‘one’, 2: ‘two’, 3: ‘three’, 4: None} |
Edit a Dict with Key, Value | a = dict.setdefault(key, value) | A. {1:”one”,2:”two”,3:”three”}, 1,”ichi” B. {1:”one”,2:”two”,3:”three”}, 4,”yon” | A. {1: ‘one’, 2: ‘two’, 3: ‘three’} B. {1: ‘one’, 2: ‘two’, 3: ‘three’, 4: ‘yon’} |
Edit a Dict with Dict | input_dict.update(pair_dict) | A. {1:”one”,2:”two”,3:”three”}, {4:”four”} B. {1:”one”,2:”two”,3:”three”}, {1:”ichi”} C. {1:”one”,2:”two”,3:”three”}, {1:”ichi”,4:”four”} | A. {1: ‘one’, 2: ‘two’, 3: ‘three’, 4: ‘four’} B. {1: ‘ichi’, 2: ‘two’, 3: ‘three’, 4: ‘four’} C. {1: ‘ichi’, 2: ‘two’, 3: ‘three’, 4: ‘four’} |
Get a Pair of Key and Value | a = dict.popitem() | A. {1:”one”,2:”two”,3:”three”} B. {} | A. {3, ‘three’} B. KeyError |
Loop with Key, Value | for key, val in input_dict.items(): print(key,val) | {1:”one”,2:”two”,3:”three”} | 1 one 2 two 3 three |
Loop with Key | for key in input_dict.keys(): print(key) | {1:”one”,2:”two”,3:”three”} | 1 2 3 |
Loop with Value | for key in input_dict.values(): print(key) | {1:”one”,2:”two”,3:”three”} | one two three |
サンプルコード … Sample Code
### Create Dict
EMPTY_DICT = {} ### dict()
INT_STR_DICT = {1:"one",2:"two",3:"three"} ### {1: 'one', 2: 'two', 3: 'three'}
INT_STR_DICT = dict(zip([1,2,3],["one","two","three"])) ### {1: 'one', 2: 'two', 3: 'three'}
### Delete Dict
### del dict
INT_STR_DICT = {1:"one",2:"two",3:"three"}
del INT_STR_DICT
### Read Length
### len(dict)
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(len(INT_STR_DICT)) ### 3
### Read Type
### type(dict)
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(type(INT_STR_DICT)) ### <class 'dict'>
### Read Value at target_key
### dict[target_key]
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(INT_STR_DICT[1]) ### one
### Edit Value at target_key
### dict[target_key] = new_val
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
INT_STR_DICT[1] = "hundred"
### print(INT_STR_DICT) ### {1: 'hundred', 2: 'two', 3: 'three'}
### Clear All Values
### dict.clear()
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
INT_STR_DICT.clear()
### print(INT_STR_DICT) ### {}
### Create Copy
### dict.copy()
INT_STR_DICT = {1:"one",2:"two",3:"three"}
copy_DICT_1 = INT_STR_DICT
copy_DICT_2 = INT_STR_DICT.copy()
### print(copy_DICT_1) ### {1: 'one', 2: 'two', 3: 'three'}
### print(copy_DICT_2) ### {1: 'one', 2: 'two', 3: 'three'}
INT_STR_DICT[1] = "hundred"
### print(copy_DICT_1) ### {1: 'hundred', 2: 'two', 3: 'three'}
### print(copy_DICT_2) ### {1: 'one', 2: 'two', 3: 'three'}
### Create Dict with constant_value for target_keys
### dict.fromkeys(target_keys,constant_value)
INT_LIST = [1,2,3]
INT_TUPLE = (1,2,3)
CONST_VAL = 0
INT_DICT_1 = dict.fromkeys(INT_LIST,CONST_VAL)
INT_DICT_2 = dict.fromkeys(INT_TUPLE,CONST_VAL)
INT_DICT_3 = dict.fromkeys(INT_LIST)
### print(INT_DICT_1) ### {1: 0, 2: 0, 3: 0}
### print(INT_DICT_2) ### {1: 0, 2: 0, 3: 0}
### print(INT_DICT_3) ### {1: None, 2: None, 3: None}
### Get Value of target_key without changing
### dict.get(target_key)
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(INT_STR_DICT.get(1)) ### one
### print(INT_STR_DICT.get(4)) ### None
### print(INT_STR_DICT.get(4,"N/A")) ### N/A
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### Get Value of target_key with changing
### dict.pop(target_key)
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(INT_STR_DICT.pop(1)) ### one
### print(INT_STR_DICT.pop(4)) ### [ERROR] KeyError: 4
### print(INT_STR_DICT.pop(4,"N/A")) ### N/A
### print(INT_STR_DICT) ### {2: 'two', 3: 'three'}
### Get Value of target_key with changing
### dict.setdefault(target_key)
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(INT_STR_DICT.setdefault(1)) ### one
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(INT_STR_DICT.setdefault(1,"ichi")) ### one
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(INT_STR_DICT.setdefault(4)) ### None
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three', 4: None}
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(INT_STR_DICT.setdefault(4,"yon")) ### yon
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three', 4: 'yon'}
### Pop Last Key-Value Pair
### dict.popitem()
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(INT_STR_DICT.popitem()) ### (3, 'three')
### print(INT_STR_DICT) ### {1: 'one', 2: 'two'}
EMPTY_DICT = {}
### print(EMPTY_DICT) ### {}
### EMPTY_DICT.popitem() ### [ERROR] KeyError: 'popitem(): dictionary is empty'
### For Loop
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
### print(INT_STR_DICT.items())
### dict_items([(1, 'one'), (2, 'two'), (3, 'three')])
for key, val in INT_STR_DICT.items():
### print(key,val)
pass
### 1 one
### 2 two
### 3 three
### print(INT_STR_DICT.keys())
### dict_keys([1, 2, 3])
for key in INT_STR_DICT.keys():
### print(key)
pass
### 1
### 2
### 3
### print(INT_STR_DICT.values())
### dict_values(['one', 'two', 'three'])
for val in INT_STR_DICT.values():
### print(val)
pass
### one
### two
### three
### Update Dict
### dict.update(new_dict)
INT_STR_DICT = {1:"one",2:"two",3:"three"}
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three'}
INT_STR_DICT.update({4:"four"})
### print(INT_STR_DICT) ### {1: 'one', 2: 'two', 3: 'three', 4: 'four'}
INT_STR_DICT.update({1:"ichi"})
### print(INT_STR_DICT) ### {1: 'ichi', 2: 'two', 3: 'three', 4: 'four'}
このプログラムは、Pythonの辞書に関するいくつかの基本的な操作について説明しています。
まず、辞書を作成する方法を3つ紹介しています。その後、辞書を削除する方法、辞書の長さを取得する方法、辞書の型を取得する方法、辞書内のキーに対応する値を取得する方法、辞書内の値を変更する方法、辞書内のすべての値をクリアする方法、辞書のコピーを作成する方法、辞書内のキーに対応する値を取得する方法(存在しない場合は既定値を返すこともできます)、辞書内のキーと値を削除する方法、辞書内に既定値を設定する方法、辞書内の最後のキーと値を削除する方法、辞書内のすべてのキー、値、およびキー/値ペアを取得する方法、辞書を更新する方法について説明しています。
Pythonの辞書について
Pythonの辞書は、キーと値のペアの集合体であり、順序は保持されません。辞書は波括弧{}で囲み、キーと値のペアはコロンで区切られます。
辞書の作成
辞書は、波括弧{}を使って作成します。空の辞書を作成する場合は、{}
またはdict()
を使用します。また、既存のリストから辞書を作成する場合は、dict(zip(list1,list2))
を使用することができます。
辞書の削除
辞書を削除するには、del
キーワードを使用します。辞書を削除する場合は、del dict_name
を使用します。
辞書の長さの取得
辞書の長さを取得するには、len(dict_name)
を使用します。
辞書の型の取得
辞書の型を取得するには、type(dict_name)
を使用します。
辞書の値を取得する
辞書から値を取得するには、dict_name[key]
を使用します。
辞書の値を変更する
辞書の値を変更するには、dict_name[key] = new_value
を使用します。
辞書の全ての値をクリアする
辞書の全ての値をクリアするには、dict_name.clear()
を使用します。
辞書のコピーを作成する
辞書のコピーを作成するには、dict_name.copy()
を使用します。
指定した値を使用して辞書を作成する
指定した値を使用して辞書を作成するには、dict.fromkeys(keys, value)
を使用します。
指定したキーの値を取得する
辞書から指定したキーの値を取得するには、dict_name.get(key)
を使用します。キーが存在しない場合は、None
を返します。
指定したキーの値を取得し、辞書から削除する
辞書から指定したキーの値を取得し、辞書から削除するには、dict_name.pop(key)
を使用します。
指定したキーの値を取得し、辞書から削除する(デフォルト値あり)
辞書から指定したキーの値を取得し、辞書から削除するには、dict_name.pop(key, default)
を使用します。キーが存在しない場合は、default
値が返されます。
指定したキーの値を取得し、辞書に追加する
辞書から指定したキーの値を取得し、辞書に追加するには、dict_name.setdefault(key, default)
を使用します。キーが存在する場合は、値が返されます。存在しない場合は、default
値が追加されます。
最後のキーと値を取得し、辞書から削除する
辞書から最後のキーと値を取得し、辞書から削除するには、dict_name.popitem()
を使用します。
辞書のループ
辞書のループには、for key, value in dict_name.items()
を使用します。for key in dict_name.keys()
を使用して、辞書のキーにループすることもできます。また、for value in dict_name.values()
を使用して、辞書の値にループすることもできます。
辞書を更新する
辞書を更新するには、dict_name.update(new_dict)
を使用します。
参考リンク … Reference Link
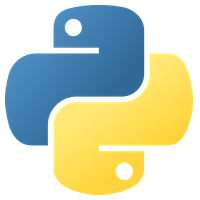
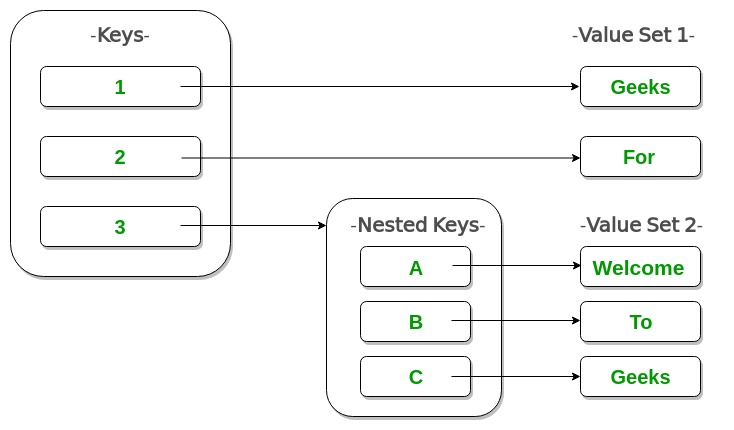