こちらは「set」(Python組み込み型)のサンプルコードについての記事となっております。
基本情報 … Basic Information
Pythonの「set」は、重複しない要素の集合を表現するデータ型です。通常、波括弧 {}
を用いて要素を定義します。
setは順序が保証されていないので、インデックスを指定して要素を取り出すことはできません。また、要素の変更も削除も可能ですが、変更することはできません。ただし、要素の追加や削除を行うことができます。
setは、集合演算(和集合、積集合、差集合など)を行う際に非常に便利です。また、データの重複を排除したい場合や、順序を気にせず要素を扱いたい場合にもよく利用されます。
チートシート … Cheat Sheet
Create a Empty Set | a = set() | set() | |
Create a Number Set | a = {1,2,3} | ||
Create a String Set | a = {“one”,”two”,”three”} | ||
Delete a Set | del a | ||
Get a Length of the Set | a=len(input_set) | A. set() B, {1,2,3} | A. 0 B. 3 |
Get a Type of the Set | a=type(input_set) | A. set() B, {1,2,3} | A. <class ‘set’> B. <class ‘set’> |
Edit a Set with Value | input_set.add(new_val) | A. set(), 999 B. {1,2,3}, 999 C. {“one”,”two”,”three”}, 999 | A. {999} B. {1, 2, 3, 999} C. {“one”,”two”,”three”,999} |
Delete Elements of Set | input_set.clear() | A. {1,2,3} B. {“one”,”two”,”three”} | A. set() B. set() |
Create a Set with Set Copy a Set | a = input_set.copy() | A. {1,2,3} B. {“one”,”two”,”three”} | A. {1,2,3} B. {“one”,”two”,”three”} |
Create a Set with Set Difference | a = src_set.difference(dst_set) | A. {1,2,3}, {2,4,6} B. {2,4,6}, {1,2,3} | A. {1, 3} B. {4, 6} |
Create a Set with Set Interaction | a = src_set.interaction(dst_set) | A. {1,2,3}, {2,4,6} B. {2,4,6}, {1,2,3} | A. {2} B. {2} |
Create a Set with Set Symmetric Diffference | a = src_set.symmetric_difference(dst_set) | A. {1,2,3}, {2,4,6} B. {2,4,6}, {1,2,3} | A. {1, 3, 4, 6} B. {1, 3, 4, 6} |
Create a Set with Set Union | a = src_set.union(dst_set) a = src_set.union(dst_set1, dst_set2, …) | A. {1,2,3}, {4,5,6} B. {1,2,3}, {2,4,6} C. {1,2,3}, {4,5,6},{2,4,6} | A. {1, 2, 3, 4, 5, 6} B. {1, 2, 3, 4, 6} C. {1, 2, 3, 4, 5, 6} |
Edit a Set with Set Difference | src_set.difference_update(dst_set) | A. {1,2,3}, {2,4,6} B. {2,4,6}, {1,2,3} | A. {1, 3} B. {4, 6} |
Edit a Set with Set Interaction | src_set.interaction_update(dst_set) | A. {1,2,3}, {2,4,6} B. {2,4,6}, {1,2,3} | A. {2} B. {2} |
Edit a Set with Set Symmetric Diffference | src_set.symmetric_difference_update(dst_set) | A. {1,2,3}, {2,4,6} B. {2,4,6}, {1,2,3} | A. {1, 3, 4, 6} B. {1, 3, 4, 6} |
Edit a Set with Set Union | src_set.update(dst_set) src_set.update(dst_set1, dst_set2, …) | A. {1,2,3}, {4,5,6} B. {1,2,3}, {2,4,6} C. {1,2,3}, {4,5,6},{2,4,6} | A. {1, 2, 3, 4, 5, 6} B. {1, 2, 3, 4, 6} C. {1, 2, 3, 4, 5, 6} |
Edit a Set with Value Remove a Element of Set | input_set.discard(target_val) | A. {1,2,3}, 2 B. {1,2,3}, 999 | A. {1,3} B. {1,2,3} |
Edit a Set with Value Remove a Element of Set | input_set.remove(target_val) * KeyError | A. {1,2,3}, 2 B. {1,2,3}, 999 | A. {1,3} B. KeyError |
Edit a Set Pop a Element from Set | input_set.pop() *KeyError: | A. {1,2,3} B. {1} C. set() | A. {2,3} B. set() C. KeyError |
Check a Set with Set Disjoint Checker | src_set.isdisjoint(dst_set) | A. {1,2,3}, {4,5,6} B. {1,2,3}, {2,4,6} | A.True B. False |
Check a Set with Set Subset Checker | src_set.issubset(dst_set) | A. {1,2,3}, {1,2,3,4,5,6} B. {1,2,3,4,5,6}, {1,2,3} | A.True B. False |
Check a Set with Set Superset Checker | src_set.issuperset(dst_set) | A. {1,2,3}, {1,2,3,4,5,6} B. {1,2,3,4,5,6}, {1,2,3} | A.True B. False |
サンプルコード … Sample Code
### Create Set
EMPTY_SET = {} ### set()
INT_SET = {1,2,3}
STR_SET = ["one","two","three"]
### Delete Set
### del set
INT_SET = {1,2,3}
del INT_SET
### Read Length
### len(set)
INT_SET = {1,2,3}
### print(INT_SET) ### {1, 2, 3}
### print(len(INT_SET)) ### 3
### Read Type
### type(set)
INT_SET = {1,2,3}
### print(INT_SET) ### {1, 2, 3}
### print(type(INT_SET)) ### <class 'set'>
### Add new_val
### set.add(new_val)
INT_SET = {1,2,3}
### print(INT_SET) ### {1, 2, 3}
INT_SET.add(999)
### print(INT_SET) ### {1, 2, 3, 999}
### Clear All Values
### set.clear()
INT_SET = {1,2,3}
### print(INT_SET) ### {1, 2, 3}
INT_SET.clear()
### print(INT_SET) ### set()
### Create Copy
### set.copy()
INT_SET = {1,2,3}
copy_SET_1 = INT_SET
copy_SET_2 = INT_SET.copy()
### print(copy_SET_1) ### {1, 2, 3}
### print(copy_SET_2) ### {1, 2, 3}
INT_SET.add(999)
### print(copy_SET_1) ### {1, 2, 999}
### print(copy_SET_2) ### {1, 2, 3}
### Create Set (Difference)
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
diff_SET_1 = INT_SET_1.difference(INT_SET_2)
diff_SET_2 = INT_SET_2.difference(INT_SET_1)
### print(diff_SET_1) ### {1, 3}
### print(diff_SET_2) ### {4, 6}
### Create Set (Intersection)
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
inter_SET_1 = INT_SET_1.intersection(INT_SET_2)
inter_SET_2 = INT_SET_2.intersection(INT_SET_1)
### print(inter_SET_1) ### {2}
### print(inter_SET_2) ### {2}
### Create Set (Symmetric Difference)
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
sym_SET_1 = INT_SET_1.symmetric_difference(INT_SET_2)
sym_SET_2 = INT_SET_2.symmetric_difference(INT_SET_1)
### print(sym_SET_1) ### {1, 3, 4, 6}
### print(sym_SET_2) ### {1, 3, 4, 6}
### Create Set (Union)
INT_SET_1 = {1,2,3}
INT_SET_2 = {4,5,6}
INT_SET_3 = {2,4,6}
uni_SET_1 = INT_SET_1.union(INT_SET_2)
uni_SET_2 = INT_SET_1.union(INT_SET_3)
uni_SET_3 = INT_SET_1.union(INT_SET_2,INT_SET_3)
### print(uni_SET_1) ### {1, 2, 3, 4, 5, 6}
### print(uni_SET_2) ### {1, 2, 3, 4, 6}
### print(uni_SET_3) ### {1, 2, 3, 4, 5, 6}
### Update Set (Difference)
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
### print(INT_SET_1) ### {1, 2, 3}
### print(INT_SET_2) ### {2, 4, 6}
INT_SET_1.difference_update(INT_SET_2)
### print(INT_SET_1) ### {1, 3}
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
### print(INT_SET_1) ### {1, 2, 3}
### print(INT_SET_2) ### {2, 4, 6}
INT_SET_2.difference_update(INT_SET_1)
### print(INT_SET_2) ### {4, 6}
### Update Set (Intersection)
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
### print(INT_SET_1) ### {1, 2, 3}
### print(INT_SET_2) ### {2, 4, 6}
INT_SET_1.intersection_update(INT_SET_2)
### print(INT_SET_1) ### {2}
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
### print(INT_SET_1) ### {1, 2, 3}
### print(INT_SET_2) ### {2, 4, 6}
INT_SET_2.intersection_update(INT_SET_1)
### print(INT_SET_2) ### {2}
### Update Set (Symmetric Difference)
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
### print(INT_SET_1) ### {1, 2, 3}
### print(INT_SET_2) ### {2, 4, 6}
INT_SET_1.symmetric_difference_update(INT_SET_2)
### print(INT_SET_1) ### {1, 3, 4, 6}
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
### print(INT_SET_1) ### {1, 2, 3}
### print(INT_SET_2) ### {2, 4, 6}
INT_SET_2.symmetric_difference_update(INT_SET_1)
### print(INT_SET_2) ### {1, 3, 4, 6}
### Update Set (Union)
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
### print(INT_SET_1) ### {1, 2, 3}
### print(INT_SET_2) ### {2, 4, 6}
INT_SET_1.update(INT_SET_2)
### print(INT_SET_1) ### {1, 2, 3, 4, 6}
INT_SET_1 = {1,2,3}
INT_SET_2 = {2,4,6}
### print(INT_SET_1) ### {1, 2, 3}
### print(INT_SET_2) ### {2, 4, 6}
INT_SET_2.update(INT_SET_1)
### print(INT_SET_2) ### {1, 2, 3, 4, 6}
### Remove target_val
INT_SET = {1,2,3}
### print(INT_SET) ### {1, 2, 3}
INT_SET.discard(2)
INT_SET.discard(999)
### print(INT_SET) ### {1, 3}
### Remove target_val
INT_SET = {1,2,3}
### print(INT_SET) ### {1, 2, 3}
INT_SET.remove(2)
### print(INT_SET) ### {1, 3}
### INT_SET.remove(999) ### KeyError: 999
### Remove Random Value
INT_SET = {1,2,3}
### print(INT_SET) ### {1, 2, 3}
INT_SET.pop()
### print(INT_SET) ### {2, 3}
INT_SET.pop()
### print(INT_SET) ### {3}
### Check Disjoint
INT_SET_1 = {1,2,3}
INT_SET_2 = {4,5,6}
INT_SET_3 = {2,4,6}
### print(INT_SET_1.isdisjoint(INT_SET_2)) ### True
### print(INT_SET_1.isdisjoint(INT_SET_3)) ### False
### Check Subset
INT_SET_1 = {1,2,3}
INT_SET_2 = {1,2,3,4,5,6}
### print(INT_SET_1.issubset(INT_SET_2)) ### True
### print(INT_SET_2.issubset(INT_SET_1)) ### False
### Check Superset
INT_SET_1 = {1,2,3}
INT_SET_2 = {1,2,3,4,5,6}
### print(INT_SET_1.issuperset(INT_SET_2)) ### False
### print(INT_SET_2.issuperset(INT_SET_1)) ### True
このプログラムは、Pythonのセット(set)に関する基本的な操作を紹介しています。プログラム内で行われている操作には、セットの作成、削除、長さの取得、タイプの取得、値の追加、値の削除、コピーの作成、差分の取得、共通部分の取得、対象差分の取得、和集合の取得、差分のアップデート、共通部分のアップデート、対象差分のアップデート、和集合のアップデート、ランダムな値の削除、部分集合の確認、上位集合の確認などが含まれます。
参考リンク … Reference Link
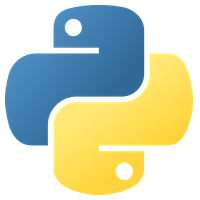
Built-in Types
The following sections describe the standard types that are built into the interpreter. The principal built-in types are...
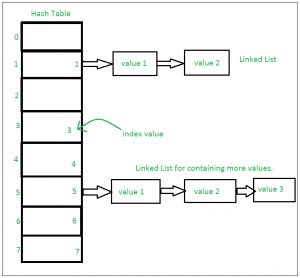
Sets in Python - GeeksforGeeks
Python sets are unordered collections of unique elements that support efficient search, insert, and delete operations, a...